Enter the height of the tree into the calculator to determine the total number of nodes visited in a complete binary tree traversal.
Tree Traversal Formula
The following formula is used to calculate the number of nodes visited in a complete binary tree traversal.
N = 2^h - 1
Variables:
- N is the total number of nodes visited in the tree traversal
- h is the height of the tree
To calculate the total number of nodes visited in a complete binary tree traversal, raise 2 to the power of the height of the tree and subtract 1 from the result. This formula assumes that the tree traversal visits every node in the tree exactly once.
What is a Tree Traversal?
Tree traversal is a process used in computer science to visit or check each node in a tree data structure exactly once. This can be done in different ways, including depth-first order (pre-order, in-order, post-order) and breadth-first order (level order). The traversal method chosen often depends on the specific needs of the algorithm being used. This process is crucial in tasks such as searching for a specific value in the tree, checking the structure of the tree, or applying an operation to each node.
How to Calculate Tree Traversal?
The following steps outline how to calculate the total number of nodes visited in a Tree Traversal.
- First, determine the height of the tree (h).
- Next, use the formula N = 2^h – 1 to calculate the total number of nodes visited.
- Finally, calculate the result.
- After inserting the value of h and calculating the result, check your answer.
Example Problem:
Use the following variables as an example problem to test your knowledge.
Height of the tree (h) = 3
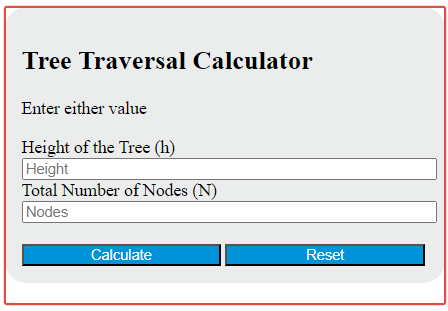